Dive Deeper into Dynamic Memory Allocation in C: Essential Techniques for Programming Success
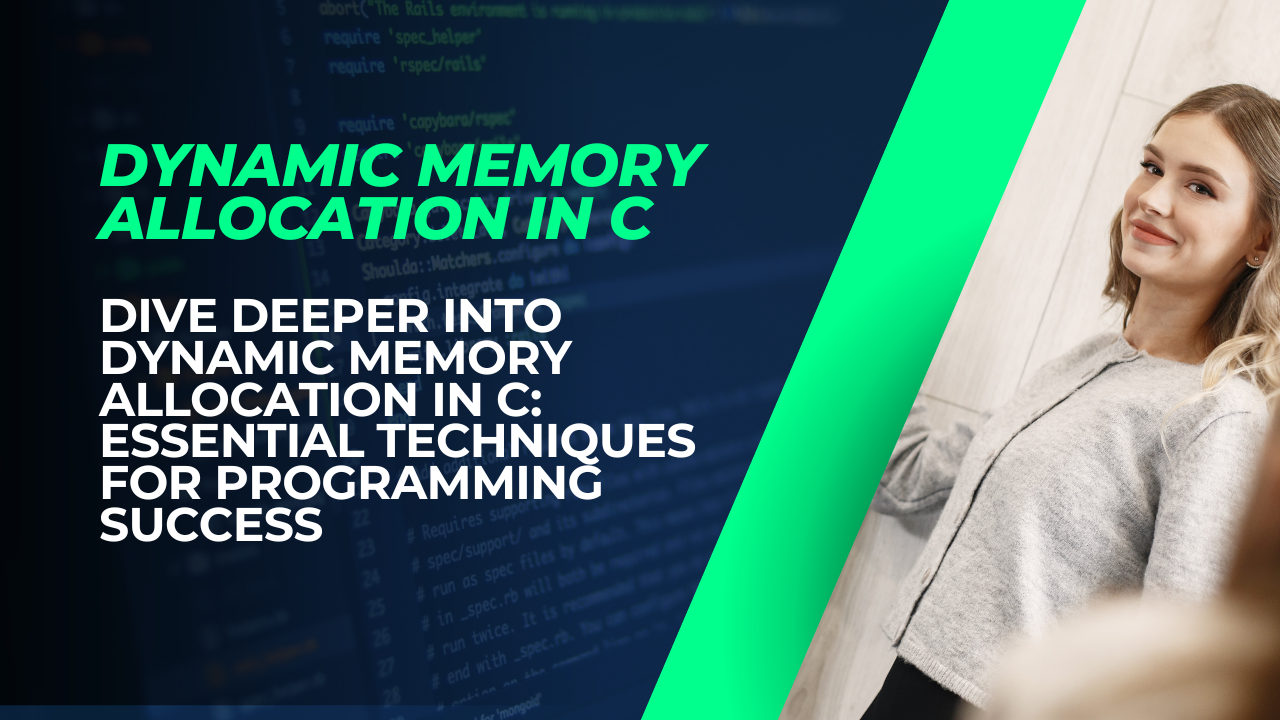
Welcome to the world of C programming, where dynamic memory allocation plays a pivotal role in optimizing efficiency and resource management. In this comprehensive guide, we'll delve into the nuances of dynamic memory allocation in C, shedding light on its significance, implementation, and best practices.
What is Dynamic Memory Allocation?
Dynamic memory allocation refers to the process of allocating memory at runtime, allowing programs to adapt and utilize memory resources efficiently. Unlike static memory allocation, where memory is allocated at compile time and remains fixed throughout program execution, dynamic memory allocation enables flexibility by allocating memory as needed during program execution.
The Basics of Dynamic Memory Allocation in C
In C programming, dynamic memory allocation is primarily achieved through three key functions:
-
malloc(): Stands for memory allocation, this function is used to dynamically allocate a specified number of bytes of memory.
-
calloc(): This function allocates memory for an array of elements, initializing them to zero.
-
realloc(): Short for reallocation, this function is used to resize the previously allocated memory block, either expanding or shrinking it as needed.
Advantages of Dynamic Memory Allocation
Dynamic memory allocation in C offers several advantages over static memory allocation:
-
Flexibility: Dynamic allocation allows programs to adapt to varying memory requirements during runtime.
-
Efficiency: Memory is allocated only when needed, optimizing resource utilization and minimizing wastage.
-
Dynamic Data Structures: Dynamic memory allocation facilitates the creation of dynamic data structures such as linked lists, trees, and graphs, enabling versatile and efficient data manipulation.
Best Practices for Dynamic Memory Allocation
While dynamic memory allocation provides flexibility and efficiency, it also introduces certain complexities and challenges. To ensure robust and reliable code, it's essential to adhere to best practices:
-
Error Checking: Always check the return value of memory allocation functions to handle potential allocation failures gracefully.
-
Memory Leak Prevention: Free dynamically allocated memory using the free() function to prevent memory leaks and conserve resources.
-
Avoid Fragmentation: Minimize memory fragmentation by deallocating unused memory blocks and optimizing memory allocation patterns.
Dynamic Memory Allocation in C Programming: Practical Examples
Let's illustrate the concept of dynamic memory allocation with a practical example:
#include <stdio.h>
#include <stdlib.h>
int main() {
int *ptr;
int n = 5;
// Allocate memory for n integers
ptr = (int*)malloc(n * sizeof(int));
// Check if memory allocation was successful
if (ptr == NULL) {
printf("Memory allocation failed");
return 1;
}
// Assign values to dynamically allocated memory
for (int i = 0; i < n; i++) {
ptr[i] = i + 1;
}
// Display the allocated memory
printf("Dynamically allocated memory:\n");
for (int i = 0; i < n; i++) {
printf("%d ", ptr[i]);
}
// Free dynamically allocated memory
free(ptr);
return 0;
}
Conclusion
Dynamic memory allocation is a fundamental concept in C programming, offering unparalleled flexibility and efficiency in memory management. By mastering the intricacies of dynamic memory allocation and adhering to best practices, developers can unlock the full potential of C programming, creating robust, adaptable, and resource-efficient applications. So, embrace the power of dynamic memory allocation and elevate your C programming skills to new heights!
- Questions and Answers
- Opinion
- Story/Motivational/Inspiring
- Technology
- Art
- Causes
- Crafts
- Dance
- Drinks
- Film/Movie
- Fitness
- Food
- Giochi
- Gardening
- Health
- Home
- Literature
- Music
- Networking
- Altre informazioni
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness
- News
- Culture
- War machines and policy